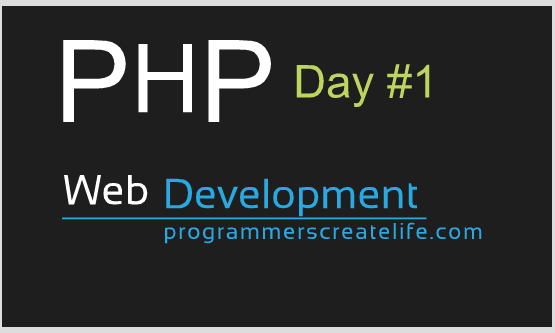
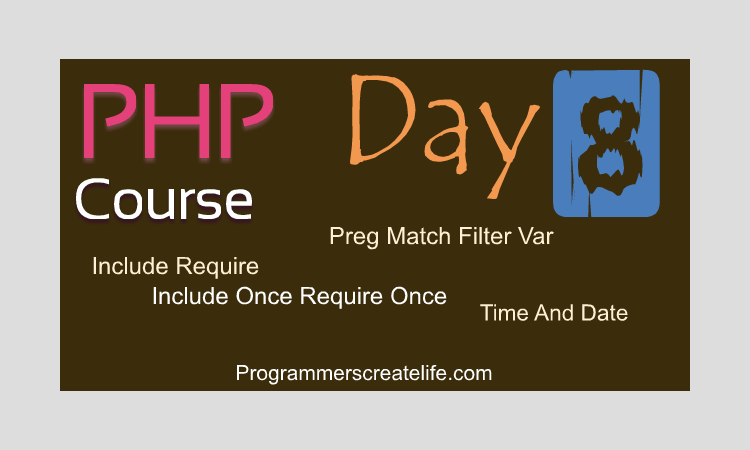
Day 8
Welcome to day8 inPHP in 15 Days – Guaranteed Training Course. Today we are going to make form submission little more secure as well as we will talk about some of really important internal functions, you must really know about. So, here is what we are going to learn today:
· Preg Match
· Include&Require
· Include_once &Require_once
· Date Handling
Preg Match:
Sometime we are in need to search something from some data no matter what type of data it is. This search can be for any purpose like security reason, want to find keywords, want to find some words to replace etc. So, preg_match() is another built in PHP function which enables us to do such kind of searches. It is kind of a search engine. We define a pattern in compliance with preg_match() rules and it searches the input data according to the defined pattern. If pattern matches within the data, it returns true otherwise false.
We have used some of internal Functions (Built In Functions) on data we received from client side. Now, we want to check the pattern and characteristics of the data. If the form data comply with our required patterns and within range of allowed characters, it will be authenticated otherwise, rejected.
Let’s try some of these patterns. You can find patterns on the internet easily. It’s better to find one and use which suits your requirements. If you want to make the patterns on your own, it’s a different subject. Now, let’s use some of the patterns which are used very often.
First of all let’s define a variable and assume this is the input we received from client side and for the user name or name where we just allow alphabets and whole numbers only.
$name = '';
Now call the internal function preg_match and between parenthesis it takes at least two arguments. First one is pattern, keep it empty for the moment, and second one is the data we want to check and match. Let’s put variable name here. The pattern here will be checked throughout the input data we’ll provide this function. If the pattern is matched within the string given, it will return true otherwise false. Let’s paste in the pattern. Here we go.
if(preg_match('/^[a-zA-Z0-9 ]*$/', $name)){
echo 'Matched';
} else {
echo 'Sorry! It does not match.';
}
This pattern is going to validate our string for small case letters froma to z, capital letters a to z and 0 to 9 whole numbers. If the preg_match finds other than defined case characters it will return false. So, for now let’s put this in a conditional statement that if preg_match finds the match and returns true then echo ‘Matched’, otherwise ‘Sorry! It does not match’
Now let’s check what we get in our browser, go to the page by typing the URL in address bar and hit enter and here we find the message ‘Matched’. It means the data we supplied matches the pattern withoutany problem. Change the value of the variable name to ‘ab’ and check this out and same result, ‘Matched’. Now let’s try to put ampersand sign in our supplied data, check for the output in the browser and you see, it doesn’t match. The reason is only ampersand doesn’t fall within allowed characters range. Let’s test for another sign and this time again it doesn’t match. So, as long as the data doesn’t match with the allowed character range or our pattern, our preg_match will supply false. Now we can check it for all character ranges, let’s put ‘abCD34’ and as names donot contain numbers, so let’s just erase the numeric or whole numberfrom allowed pattern, from here and let’s go back to the browser. Again you can see it doesn’t match as we changed the range. This is how we bound our users to use only the letters or number we allow.
Filter_Var:
Now let’s move further and validate email addresses. For this purpose PHP provides a very good internal function filter_var. Let’s create a variable email first and just keep it empty for now. Let’s use the internal function filter_var() This function takes two arguments. First one is data and second one the type of filteration. Let’s put email here and tell it the filter option that validate email. Now let’s put a dummy email address here ‘mick@lazygag.com’ and you can see it’s a valid email address.Let’s just echo this.
$email = 'mick@lazygag.com';
echo filter_var($email, FILTER_VALIDATE_EMAIL);
This function returns the data if it’s valid otherwise returns false. Here we go the email address is shown, it means it’s a valid email address. Now, just change the @ sign of email with # and make it invalid. Go back to the browser and you can see no output means it returned false. Now try tosupply two @ signs in email address which is again invalid. Check this out in browser one more time and you can see no output. So, this is how you can easily validate user defined email addresses.
Now let’s move a little further and use preg_match again to validate URLs supplied by users. Define a variable URL and keep it empty for now.
$url= '';
Now let’s call the preg_match function and keep empty the pattern, that we’ll just paste one in a moment and supply the second argument i.e. our variable which will store incoming URLs. Now let’s paste the pattern here which is going to validate any type of URL.
preg_match('/^(http|https):\\/\\/[a-z0-9]+([\\-\\.]{1}[a-z0-9]+)*\\.[a-z]{2,5}'.'((:[0-9]{1,5})?\\/.*)?$/i', $url);
Now let’s enclose it in a conditional statement as we did before and if it matches echo ‘URL matches.’ Otherwise echo ‘Sorry! URL does not match.’.
if(preg_match('/^(http|https):\\/\\/[a-z0-9]+([\\-\\.]{1}[a-z0-9]+)*\\.[a-z]{2,5}'.'((:[0-9]{1,5})?\\/.*)?$/i', $url)){
echo 'URL matches.';
} else {
echo 'Sorry! URL does not match.';
}
Let’s run this in the browser and here you can see URL doesn’t matches as we did not supply any URL. Now, let’s go back and supply URL.
$url= 'http://www.lazygag.com';
ThisURL is really a valid URL and it must be validated if our pattern is fine. So, let’s check this out and you can see it’s valid. Let’s put a br tag between messages to make these more readable.
Now if we remove http:// and check it for its validation, and you can see it doesn’t match. It means our users need to supply http:// or we can append it after getting URL from user. Let’s put http back in place and append with www. And check this out and it matches. Now if we put any character within URL which makes it invalid our preg_match will find it out. Let’s try couple of those andyou can see each time we’ll get invalid message. This is the way we can make our site more hackers proof and secure. So, each time you get data from user side (client side)you must validate it properly.
Include & Require:
Very often we need to merge one file in another file. So, to combine two files we use include or require. When we include a file in another file these two act and perform like one file. Include and require have same meaning as these meant in our day-to-day English. Practical demonstration would be better to understand this phenomenon. Let’s do this.
Here we have a very simple HTML page having HTML and CSS code. Here is head section and below here is body area. Within body section we have three divs. First one is having header section and below here is footer and between two of these we have our content area having id ‘content’. Above here in head section of HTML we have written some style. Now, let’s take a look at this page in our browser. So, this is really a simple page just for demonstration purpose only. Three sections separately.
<!DOCTYPE html>
<html>
<head lang="en">
<meta charset="UTF-8">
<title></title>
<style>
#header {
background-color: darkkhaki;
height: 100px;
width: 100%;
}
#content{
background-color: antiquewhite;
height: 200px;
width: 100%;
}
footer{
background-color: darkkhaki;
height: 100px;
width: 100%;
}
</style>
</head>
<body>
<div id="header">
<h1>This is body Area</h1>
</div>
<div id="content">
<h3>This is content area.</h3>
</div>
<footer>
<h5>This is footer area</h5>
</footer>
</body>
</html>
Now we are going to break this page in parts and will make different files of this one page. Let’s create our first file i.e. ‘header.php’. I am going to cut all the code which has the head section of HTML and portion of body which is going to repeat on each page of our site, just assume. Div having id header is also going with this code to our new file header.php. Just cut it and go to our new page and here first close PHP tag as we need to paste all HTML code here and past thatin here. This is header.php file and we will use it in a moment.
//File: header.php
<!DOCTYPE html>
<html>
<head lang="en">
<meta charset="UTF-8">
<title></title>
<style>
#header {
background-color: darkkhaki;
height: 100px;
width: 100%;
}
#content{
background-color: antiquewhite;
height: 200px;
width: 100%;
}
footer{
background-color: darkkhaki;
height: 100px;
width: 100%;
}
</style>
</head>
<body>
<div id="header">
<h1>This is body Area</h1>
</div>
Now, let’s create another file and name it footer_file.php. This is the file which will contain all the footer section which will remain same for all of the pages of our site. Let’s just cut it from here and on the footer_file.php first close the PHP tags and paste all the code here and that’s it.
<footer>
<h5>This is footer area</h5>
</footer>
</body>
</html>
In the index.html file, you know we cannot write PHP code as that wouldn’t work. So, let’s rename it and change it’s extension from HTML to PHP.
Index.php
Now we have three files recently created, index.php, header.php and footer_file.php. As we brokethe main file.Let’s check out index.php file in browser. And now you can see only content area is there, i.e. the middle div having id content, also there is no styling or css at all. This is because we have removed head section which contains our CSS code, along with first div having id ‘header.’ Now we are going to combine all of these three separate files again.
Here we need to call the internal function ‘include’ and between parenthesis and between quotes write the file name you want to include. As this file is in same directory where the index file is, so we are just going to type its name, and that’s it.
include('header.php');
Let’s try this in our browser and here you can see the styling is back and ‘This is body area which we have written in our header div is back. If we look at our source code, it’s same like before as it was one file once. Everything is available now as one file, HTML head section, header div and content div etc. Now we can do same action with our footer_file and include it too. Let’s include it now, open and close PHP tags and between these include ‘footer_file.php’.
include('footer_file.php');
Let’s go back to the browser and here we go, our footer area is also back. Let’s take a look at source code again and you can see this file is now one complete file having all the sections. So, this is the way we include files and make those a part of current file. One thing you need to keep in mind that define the exact path to that file you want to include. If you give a wrong path where file doesn’t exist you will get an error.
include('../header.php');
Let’s change the path for our header file a bit this ‘../’ means look in directory one level up. Now check this out in the browser and you can see the error message.Here you can see that we have complete output except the header section. We have our middle section as well as footer and an error at the top. Now let’s just change the ‘include’ to ‘require’ and let’s see what happens now.
require('../header.php');
You can see error messages are there same as before but below you can see no output. Content and footer areas are also missing. So, this only one big difference between include and require. Try to figure out which files you want to include and which you should require. All those files on which most of the page code depends you must always require them and for all those files which are not very necessary and in some case where you consider if those fileswouldn’t run somehow, the other code should work, use include. We mostly require database files as all the information we display on pages mostly come from database. Now let’s correct the path and go back to browser and you can see everything is fine.
require('header.php');
Now, let’s move our header and footer_file to another directory and name it as includes. Now go back to the browser without changing the path and check this out you can see our script couldn’t find the files here in day8 directory and halts the script as we are requiring the files. As we have made another directory in this same directory where the index file resides, so, let’s type the name of directory and then file name. This will work now. And same action we need to take for footer_file as well. Let’s fix that too, and here we go our footer is back again.
Now one thing more we want to do here,besides putting just the path and file names we can define the exact path to this folder where this current file resides by using ‘__DIR__’ and then define the path of the file we want to include. What this will do for us, the script will not take long to search the files we need to include. It would exactly know where the file is and will include that promptly.So , dir, defines the full path to the current directory. And we need just one slash before the file we already defined.
include__DIR__ .('/includes/header.php');
Let’s go back to our browser, refresh and you can see no change here. Just remember of this slash here. Also, you can do this without these parenthesis and can also put parenthesis around this code. Like this.
include(__DIR__ . '/includes/header.php');
Let’s do this for footer as well. __DIR__ and path of the file also don’t forget to include slash here,
include(__DIR__ . '/includes/footer.php');
Let’s just remove to check what happens if we don’t put it. Refresh the browser and you can see file not found error message again. So, put the slash back and now it is working fine.
Now we know how to include and require files and how we can require/ include any file anywhere we need.
Let’s create a new file ‘function.php’ and in this file I am going to create just one function and name it as sanitize. This is the same function we created before and you already know it. Just define one argument and that is ‘data’ and inside curly braces here, let’s use our three internal function in one line, trim, ‘htmlspecialchars’ and ‘stripslashes’, you can do this in this way too. Put our incoming data into it and store it in a variable called data too. Now let’s just return this data as we need perform more processing on it.
//function.php
functionsanitize($data){
$data = trim(htmlspecialchars(stripcslashes($data)));
return $data;
}
This is our file containing only one function. Let’s get back to our index file and little bit down here, assume we need to use that function we just defined. Let’s create a variable ‘name’ and assume we are receiving data of a user form and now we need to sanitize it.
$name = 'Lazy Gag';
I want you to guess what we should do here to use the function we created in function file. Let’s call this function that we don’t have in our index file and try to sanitize ‘name’.
echosanitize($name);
We know that it wouldn’t work as we did not define this function here in this file. Refresh the browser and you can see ‘call to undefined function’ becauseourscript doesn’t know about this function. Now to have this function in this file, let’s require function file. As soon as we require the function file the ‘sanitize’ function is available on this file to use.
require(__DIR__.'/function.php');
Refresh the browse and the error has gone. Let’s just echo the sanitized name to confirm our function worked. And here we go, you can see the name. So, this is all for include and require.
Include_Once & Require_Once:
Include_Once and Require_once are same like include and require. Only the difference is, these include the files once in the whole script. It might happen that by mistake we sometimes try to include a file which we already have. Like a database connection file, just for instance. The second time when we call include_once or require_once, our script will include this only if the file has not already been included.
Let’s try this now. Here we have our previous index.php file which has already header, function and footer files required and included. Now let’s try to require our header file one more time.
require(__DIR__.'/includes/header.php');
Let’s just copy and paste it in there. Let’s go to the browser and you can see it’s required two times. Now, let’s just change require for this file only to require_once and view it.
require_once (__DIR__.'/includes/header.php');
You can see this is required only for one time. You should use this functionality where you want some specific file included only for once for one whole script. Include_once also behaves same like require_once, let’s change these to include_once.
include_once (__DIR__.'/includes/header.php');
And just try it one more time, refresh and no change at all. Now let’s change these back to include and cut one included header file from here and paste it way down below. This is the thing you might need sometimes so, you can include one file for several times, anywhere you want. Also, if you want this file only once in your whole script you can simply change it to include_once and it will be included once only in the whole script and this is all for include_once and require_once.
Date Handling:
Date and timeare very important parts of any programming language. Very often developers need to create logic as well as actions taking date/time in consideration. Also we often use it to store it in database to keep record of the information of transactions and storage history timings and for retrieval of data according to date and time. Also, we need it mostly when we develop applications in which different events fire according to date and time, like admin panel, inventory system, a booking or analytics display program etc. So, date and time are very important part of programing. Now let’s see how we can get the date.
Grabbing the date/time is a very simple and easy task. There is an internal function in PHP called date. So, we use it to grab date. So this is our date function. It takes two arguments.First one is compulsory where we tell date function the format of date and what we need as a date, second argument is optional, we’ll talk about that later in this tutorial.
Now, let’s provide our first argument between single or double quotes to this function. Let’s put ‘y’ in here and this y will show us the last two digits of current year. Just echo it out.
echodate("y");
Check this out in browser and you can see it’s 15 the current year 2015. Now, let’s just change this small y to capital Y which will display year in 4 digits. Check it out and here we go 2015. You can also put different notations like –(Hyphen) or ,(comma) and or /(slash) or put spaces between. Now, let’s put m with our Y and join them with a hyphen. Here you can see the current month in two digits along with current year in 4 digits.
echodate("m-Y");
Let’s try another one that is d and it will display current day of the month in two digits. You can change the sequence that suits your needs and date will become accordingly. If you use capital D, this will show the current day of month in letters containing first three letters only. If you use l(Small letter of L) it will show the full name of the day, here we go it’s Tuesday. Now, let’s change m to capital M.It gives us first three letters of the name of current month. Let’s format it a bit to make it more readable. You can do anything you want with this format.
echodate('l d M Y');
Let’s change it back to normal date.
echodate("m-d-Y");
Now let’s look at another thing and that is mktime(),
echomktime();
This functionreturnsUnix timestamp and displays the number of seconds from January 1st1970 to the current date. We can display it by just calling this built in function and just echo it out put a br tag between the times to display these line by line. So, here you see the number of seconds and these are from January 1st 1970 to this current time.
There is another internal function time() which serves same like mktime, number of seconds from January 1st1970. There is a little difference between time and mktime. We can supply arguments for mktime function but time doesn’t accept any arguments. These arguments tell mktime function a specific date and mktime returns number of seconds till that date from January 1st 1970. All the arguments are integers and first one represents hours, second one is minutes, third seconds, then month, then day and then year.
mktime(0,0,0,11,5,2011);
So, this argument is dictating mktime to provide the exact number of seconds to this supplied date. So, here we are going to grab the number of seconds from 1st January 1970 to 5th November 2011.We could add hours, minutes and seconds too but we kept them to 0. Now here you can see the big difference of seconds displaying our own defined date and to current date.
Now let’s store this in a variable and name it defined_date. Now what we are going to do is, we will convert thesenumber of seconds in to a formatted date using our date function. So, first argument, just define the format in which we need the output, and here is the second argument which is the number of seconds since 1970. Let’s put our variable defined_date here as a second argument. Let’s just echo it out and also put a br tag here too.
echo$defined_date = mktime(0,0,0,11,5,2011);
echodate('m-d-Y', $defined_date);
Go to the browser, hit refresh,you can see this is the exact date which we defined in mktime function, 11th month, 5th day and 2011. So, the second argument can be a time stamp or any string which denotes a date.
We can manipulate dates and we can add or subtract duration of time from the date to get some other dates. Like assume we want the date of day after one day or after seven days.We can get that easily. There are lots of ways you can achieve this result but for now let’s do it in our own way. Let’s call mktime() function and put a plus sign means we need to add time. As you know, mktime returnsnumber of seconds, so we can add seconds in this. Let’s assume we want to add one day in current time. To do so, we need to add seconds,equivalentto one day, so, let’s convert one day in seconds. 1 , i.e. 1day, multiplied by 24 i.e. the hours in one day, multiplied by 60 i.e. minutes in one hour and multiplied by 60 i.e. seconds in one minute and that’s all.
mktime() + (1*24*60*60);
Let’s store these total number of seconds till tomorrow in a variable and name it tomorrow.
$tomorrow= mktime() + (1*24*60*60);
Now we need to present itas aformatted date, so,let’suse theinternal function date. Let’s just put D in the function as first argument which will show us name of the day.Now let’s just putvariable tomorrow as the second argument here.Just echo it out and let’s just get back to the browser to view the result.
echodate("D", $tomorrow);
Hit refresh and you can see it’s Wed, which isactually tomorrow and right now it’s Tuesday here. Now, let’s convert the formatto display full date by putting m-d-Y. And here we go, it shows 12th which is the date tomorrow.
echodate("m-d-Y", $tomorrow);
Now to add 7 days in our current time and date just put 7 instead of 1, here, and go back to the browser, hit refresh and you can see 7 days have been added to the current date.
$tomorrow= mktime() + (7*24*60*60);
You can do same thing with time, instead of mktime and for now there would be no difference at all.
In PHP there is a function which converts string to time. Using this we can change any reasonable string pointing to dates can be converted to readable dates. Let’s create a variable ‘user_defined’ and store a string in it.
$user_defined = 'Sunday, 26th July 2015';
Let’s check a correct back date and it was 26th on Sunday July and 2015. You can see this is a pure string. Now let’s convert this string to time. Strtotime() is an internal PHP function let’s just call this and store result in a variable timestamp .
echo$timestamp = strtotime($user_defined);
Just echo it out. Hit refresh and you can see, our PHP has converted it to seconds till that date. Now we can convert this back to formatted date. Let’s use the internal function date() and define the format werequire and put this timestamp variable as a second argument.
echodate('m-d-Y', $timestamp);
Let’s check this out in the browser and here you can see 11th month, 26th day and 2015. Below here you can see this we have in our calendar 26th and Sunday and 2015. So, in short we just got some string which might have date in words like this and converted it to time which produced seconds and then we formatted it to our required format. There are lotsof things you can do with date and time and there are lotsof notations you can use for different kinds of date formatting. So, I recommend you to visit php.net . Here you can see lots of functions dealing with date and time.You can take a look at these but don’t let yourself confuse. Right now I just want you to explore date function thoroughly so just write it in the search box and find it. Here we are, at this page, just go through it and you will find yourself comfortable using date function and using it in your projects.