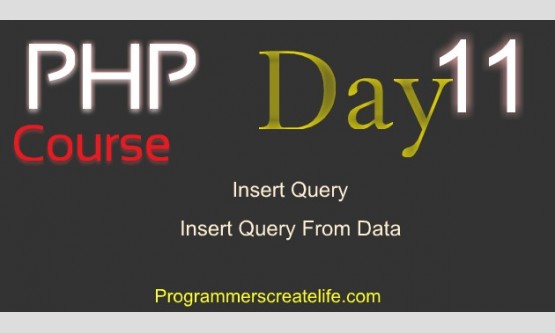
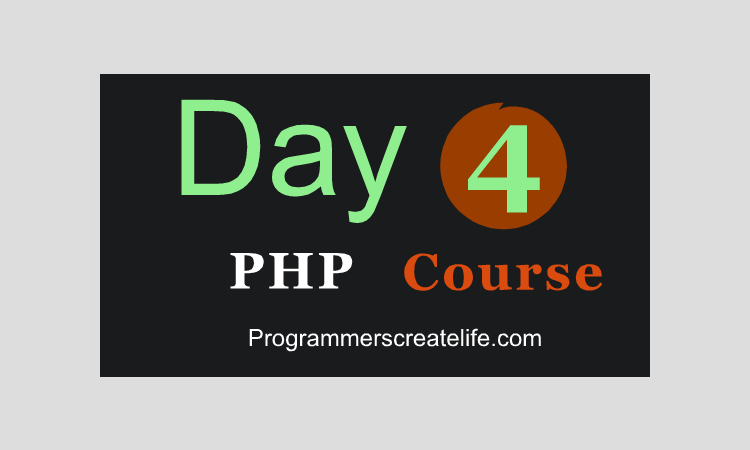
Day 4
Welcome to Day4 in PHP in 15 Days – Guaranteed Training Course. Today we are going to study about loops. What are loops and what these do for us?
· Loops are primarily used to perform a specific task for several times.
· Loops are meant to loop through(iterations).
· Every Loop depends on conditional statements. Until and unless conditions remain true, loops keep on looping through.
· A loop starts from a particular point and check some condition, if condition is true it completes its first iteration or circle and then comes to starting point. Again checks for true condition and if its, continues to second iteration and so on. The time condition returns false the loop stops immediately.
·
All most all the loops behave same, except
Foreach Loop which we will study in a future lesson.
There are several types of loops and these are:
1.
For Loop
2. While Loop
3. Do-while Loop
All of the loops are almost alike, except few differences. Let’s have a look at for-loop syntax.
For Loop:
for(definevariable; condition; control-iteration){
code statement execution;
}
First of all write the keyword for and then start parenthesis and between these we define three things.
1. A variable to start and control iterations. This variable is used to control the iterations.
2. Condition to be met for iterations. This condition is responsible for the loop to keep looping and stopping the loop.
3. Lastly the increment or decrement of the variable so that loop might stop at some point and don’t become an infinite loop. (An infinite loop will overload and halt your server so use your head )
Now start curly braces and between these you can write all of your script or code you want executed on each loop or iteration.
Now, let’s create our first for loop.
for($x = 1; $x <6; $x++){
echo $x, '<br>';
}
Let’s start for loop and define a variable x and let’s make it equals to 1. Now we need tocreate a condition which must stay true for the times we want to run this loop. Let’s say until and unless the x is less than 6 keep looping through. So, every timeiteration is completed this condition is checked and if true loop will continue. Now we have all set up but we have an infinite loop right now as we haven’t yet defined the increment.So, let’s increase variable xat the end ofeach loop. $x++ .Now, we’ve all set up, let’s output this code and here we go loop runs for 5 times and as soon as x becomes equalto6 loop stops, as condition to stay less than 6 returns false.
You can write any code within curly braces and it doesn’t matter what you do or want to do. Even you can put your most complex code here. Just for demonstration let’s output the value of variable x. Just echo it out and append it with a br tag so, each value of variable x stays unique line.
for($x = 1; $x <6; $x++){
echo $x, '<br>';
}
So, this is how we create and run for-loop.
There are some keywords we can use to stop the loop for some reason, or skip some iteration for some reason.
1. Break
2. Continue
Break:
Whenever a loop finds the keyword‘break’, loop immediately stops and will absolutely do not iterate further. No matter the condition is still true. So, this break keyword is used to stop the loop immediated. Let’s have a look how we use this keyword.
for($x = 1; $x <6; $x++){
if($x == 4){
break;
}
echo $x. '<br>';
}
Let’s create the same loop we created before and within the execution area we need to define a conditional statement. Let’s create one now. If our variable x has the value 4 only then break out of the loop. What this condition will do, it will check for the value of x each time and whenever it will find x equals to 4, the statement will execute and there is ‘break’ keyword which will stop the loop. Let’s execute it and here we go the moment x became equals to 4 loop broke and couldn’t execute further. We can make sure by just echoing the variable x after and beyond the loop, what the value x has right now.
echo$x;
You can see variable x has the value 4. Wecan also just put use break within the loop and it will prevent the loop from running all-together.
Continue:
Continue is another command you might find very useful for one of your projects. It’s very interesting. Whenever continue is found within the loop, the concerned iteration is skipped and loop jumps back to starting point for next iteration or loop.
for($x = 1; $x <6; $x++){
if($x == 4){
continue;
}
echo $x. '<br>';
}
Here we have the same code except break keyword, we are using continue. Let’s execute it. You can see that 4 is missing but 5 is there. So, it’s obvious that loop didn’t executed the statement when x became 4 and jumped to next iteration. As we have the conditional statement, if x becomes equals to 4 only then ‘continue’ keyword will be executed.The loop completes the iteration for the given number.
These are few basic examples of using break and continue only for demonstration purposeswhilewe can do really complex things while creating tables, getting data out of databases or while calculating different scenarios. I recommend you to read more about for-loop, break and continue at php.net. Also, you will become familiar and comfortable with these after using these once or twice within your professional projects.
While Loop:
While loop functions almost like the for-loop. There is only a minute difference in the way of declaring the arguments on different places.
Let’s have a look at the syntax of while-loop.
variable;
while(condition){
code statment execution;
//at the end of loop
control iteration;
}
First of all we need to define variable outside the while-loop. So, it’s a plus point that you can use any variable from your external code whether it belongs or doesn’t belong to while-loop. So, very often this loop is used to extract data from database results. We’ll learn about this in a later chapter. After declaring while loop starting point with‘while’ keyword start parenthesis and between these we just define a condition. As far as the condition stays true while loop keeps on iterating.Now start curly braces and write your code which will produce the output. Now just before the closing curly brace make increment or decrement to stop this loop, so we don’t run an infinite loop. And that’s it. You can see yourself that all the arguments are same just like for-loop except for the placement.Now, let’s create a very simplewhile-loop. I am going to create the same loop as we did before in case of for-loop. Also, we shall get the same output from our while loop.
$x = 1;
while($x <6){
echo $x, '<br>';
$x++;
}
echo 'X is : ', $x;
Declare a variable x equals to 1 and this is the variable we’ll use in our while-loop. Now start loop by while keyword and let’s create a comparison statement x < 6, i.e. loop will run till x is less than 6. Just echo the value of x and put br to show eachvalue of x line by line and at the end let’s just increment x by one at each iteration to stop the loop. OK. We are done here just take a look at the output. And there we go, same output as we hadfrom the for-loop. If we echo x now its equals to 6. Just put a string,‘x is’, to make it more clear. So, that’s our while loop. Now move on to the do-while loop.
Do-While Loop:
Do-while loop is exactly same as while loop with no differences except the execution is before the condition is checked. As its obvious from its name do-while(Like I say, do something before while condition is checked). This loop will take its first iteration,no matter, whetherthe condition is true or false.
$x = 1;
do{
echo $x, '<br>';
$x++;
} while($x <6);
echo 'X is : ', $x;
Create same like while loop and reverse its order. Write the keyword do and curly braces and everything we created for while loop and at the end put while keyword and between parentheses put the condition. We can use x previously defined but at this point x is equals to 6 so, the loop will run once only as we discussed it before although the condition is false. But let’s just comment while loop to keep x equals to 1 to use in do-while-loop. Let’s go to the browser and refresh, you can see, same output.
Now to show you the difference between while and do-while, let’s make the condition false right from the beginning, let’s append the condition x less than 1 and variable x equals to 1 as well.Now we want to run our loop until variable x is less than 1. But we have declared x equals to 1. So, let’s have a look at the output. You can see its outputting the result of its first iteration by echoing x. It means it did loop through once and value of x is now 2. So, if you need the functionality something like this where you want to run the loop at least once regardless of the condition true or false, you should opt to use do-while loop.
This is all for loops as well as for day4. I hope you will not jump to day5 without doing enough practice of loops. Take care.
Assignment:
Write a script which runs once and produce the following;
1. Displayonly even numbers on each line.
2. Do not show numbers like 10, 20, 30 and so on.
3. Do not go beyond 200.