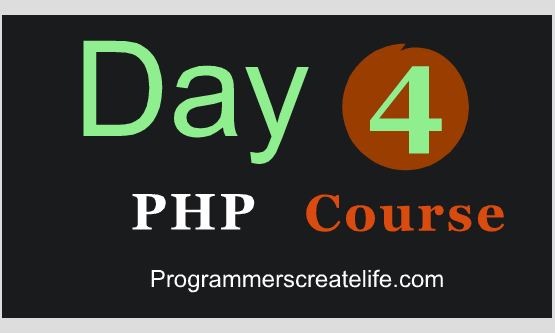
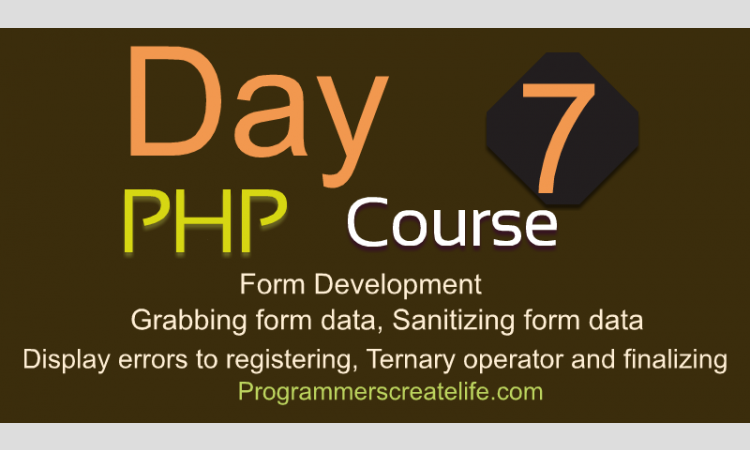
Day 7
Welcome to day7 of PHP in 15 Days – Guaranteed Training Course. It’s going to be really interesting day today. We’lllearn many interesting,extremely important and frequently usedfunctionalities. So, here is today’s schedule:
· Form Submission
· Getting & Sanitizing Form Data (Security Concerns)
· Display Required Field Errors (Form filling errors)
· Handling Form Data
Before starting today’s session, I expect that you have thoroughly gone through everything we studied on day6 and now you arefairly familiar with get, post and request super-globals as well as method and action attributes of a form. If that’s not the case I recommend you to go back to training session of day6 and try to absorbeverything we discussed there and practice it well.What are we going to study today heavily depends on tutorials of day6.
Form Submission:
Let’s try to understand, how important role a form can play in websites. There are many survey sites that collect views and reviews of public and this is possible for them only because of forms. Many emailing sites like gmail, yahoo and Hotmail etc, can register users and let them login only through forms and form submission. Every social media web-site have forms that let you submit any of your documents, information, photos, videos or any other stuff. Any site that tends to facilitate every user with everyone’s special needs and keeping privacy intact, requires form to register and login and then to communicate with you as well. So, you see, forms are everywhere. Article writing and posting them is also a form of form submission. So, there are millions of forms everywhere on the internet. You cannot even count them. Forms provide huge support to make websites dynamic. It’s the largest way of data transfer within web-applications. So, you can guess now, how important the forms are.
Now, what we want actually is, with this functionality, we will keep our website online. When a user will come to our site, let’s suppose, he wants to register with us, he will fill this very form and when he will click submit button the data will be processed the way we defined it.
Now what we are going to do here is just make a form and submit it and then grab the values of form submitted. For now we will just echo these values. Let’s make our form. This is purely HTML and you must know, how to do it. Start form tag and put the method post as we are going to submit our data by post method and define the action, on which file you want to receive the data of this form. We can submit the form data on this page too by just putting the name of this file, or if we leave it blank it means the form data will be submitted to this page too. Now just close the form tag. Now we need to create some of the input fields for users to type in their data. So, think of what the information we might need to register a user. Let’s create our first input field and keep its type text and name it as name as we need user’s name.We have already discussed about this name attribute in our day6 training session, if you don’t remember please go to day6. Let’s give a heading of ‘Registration Form’ and let’s put the placeholder for this field ‘Full Name’. Make another input field, declare type of this again text and name it as ‘username’ and placeholder as ‘User Name’ . Another one, give type as ‘email’ and name it as ‘email’ and placeholder ‘E-mail’ as well. As this is going to be the ‘User Registration Form’ so, user needs to set a password too. For this we need to create two input types. First input and type of it ‘password’ and name it as ‘password’ and placeholder ‘Password’ as well. The second field of password is to verifywhether user knows what he typed or made a mistake.So, input , type ‘password’ and name of it ‘password_again’ as we need to keep its name changed from the above input field for password and let’s just put a placeholder and make it little different too i.e. ‘Repeat Password’. Finally we need a button tosubmit this form, so, just define another input and the type is ‘submit’ and let’s just give it a value that will be shown on the button ‘Register’. One more button which can be used for user-friendliness only and that is to reset the form values. Let’s make it, input, type equals ‘reset’ and value equals ‘Reset’. This is our form and it’s sufficient for now for demonstration purposes, there can belot more fields like contact number, address, or gender, country etc, but for the moment we need only this registration form. Put ‘br’ tags to make our form look little nicer.
<h3>Registration Form</h3>
<form method="post" action="form_submission.php">
<input type="text" name="name" placeholder="Full Name"><br><br>
<input type="text" name="username" placeholder="User Name"><br><br>
<input type="email" name="email" placeholder="Email"><br><br>
<input type="password" name="password" placeholder="Password"><br><br>
<input type="password" name="password_again" placeholder="Repeat Password"><br><br>
<input type="submit" value="Register">
<input type="reset" value="Reset">
</form>
And this is it, here you can see we have created our HTML form which is fully ready to send user’s data to us and we can grab it very easily using one of our super-global $_POST as we have set the method ‘post’ in our form.
Now let’s code to grab the data of our form which will be submitted by the site visitors. Here, instead of keeping action attribute empty of our form you can define the file name where you want to submit data of this form. Let’s put the file name of this file though you can keep it empty.
action="form_submission.php"
Getting Form Data (Grabbing User Submitted Data):
So, let’s start writing the code now for grabbing the data. First thing we need is, our code should not through any errors if it doesn’t find the array that is $_POST (super-global Array). So, let’s start an if statement and put the condition if $_POST, it means we are defining if the conditional statement finds the $_POST array then carry on other wise don’t
if($_POST)
Now in the execution area let’s just echo ‘Form submitted.’
echo'Form submitted';
So, here, when a user will submit the form, no matter he fills it or not, but just submits the form, our code will show the message ‘Form submitted’. Let’s just verifythis by submitting the form.
if($_POST) {
echo 'Form submitted';
}
Here we go, just submit the form and we get the message. It means the form is being submitted without any errors and user will get this message, if one submits the form. Here I just wanted to show that when the form is submitted only then our code runs and does something and therefor it will not throw any errors now.
Now let’s create the actual code to grab the form data. To grab data we are just going to use post super-global and put the name within square brackets and echo it out.
echo$_POST['name'];
Now just refresh the browser and there is nothing right now but as soon as a user fills the name and submit the form we will see user’s input displayed. Let’s put some dummy data here in this field ‘Full Name’ and you can see the same output here as we grabbed submitted data and echoed it out.
This is how we simply can grab all the data, user submits to us. Now, let’s grab all of it and for now just echo all the data. Just copy $_POST[‘’]; and paste it several times to save our time and now just put all the values of name attributes, we defined while creating our HTML form. ‘username’, ‘email’, ‘password’ and ‘password_again’.Let’s just copy and paste it. Remove this extra one and echo all of these.Now we have written the code to grab data of every field of the form.
echo $_POST['name'];
echo $_POST['username'];
echo $_POST['email'];
echo $_POST['password'];
echo $_POST['password_again'];
Let’s go to the browser now. Refresh it and let’s just put some dummy data in all the fields, hit Register and there you go, you can see all the data input by a user at the top as we just grabbed all the data from the form and echoed it out.
Let’s explain the whole scenario. When a user will come to our site he will find this form for registration. He will fill all the fields of this form and will submit it to us. Now, when form is submitted we have placed the condition to run our PHP code only at the time when form is submitted. Afterwards, we grabbed all the data and we can do anything we want with this. Usually we store this data in our database and thatis what we will do withinnext couple of days training sessions. But here we just echoed all the data we grabbed for demonstration purposes only.
Sanitizing Data:
It’s always really important to keep in mind that any data which you accept from user’s side must be thoroughly checked and sanitized for security purposes. As we mostly store the user submitted data in our data base. So, if we don’t sanitize it the user may be a hacker and can send special characters and or queries to damage our whole database as well as can get the information of the structure of site and can easily hack our whole site. So, very basic things we must consider, we will do in this tutorial.
Here we have our same form we created just before and same code for grabbing, user input form data. Now we need to sanitize it to make it a secure form submission.Let’s just do it.
We have some internal functions (built in functions) which we can use to easily escape special charaters, trim the extra spaces and get rid of special HTML characters. So, first one is trim, this function trims all the extra spaces used within the input supplied by the user. Let’s just cut the input and paste it within this function.
trim($_POST['name']);
Now we want to use the trimmed version of the input so let’s just store this in a variable of our own and name it as $name.
$name = trim($_POST['name']);
Now we are going to use another function ‘htmlspcialchars()’ and what this is going to do is it will clear the input from all type of special HTML characters. Now let’s perform this function on our trimmed version and not on the direct value from the form. As if we will use it on directly grabbed field data it wouldn’t be trimmed. So, use the trimmed data in this step.
$name = htmlspecialchars($name);
Now the third step is to call another internal function ‘stripslashes()’ and use this function on our trimmed and free from html special characters data. Put name in the parenthesis and that’s it.
$name = stripslashes($name);
So, now we have sanitized and drained our input ‘name’ successfully. So, this input is free of extra spaces, free from HTML special characters and free from the slashes now, which can help defend hackers’ activitiesup to some extent.
Now this is time to perform whole of this process again on all of our input data one by one. We need to trim, make it free from special characters and slashes. So, as we need to perform the same task several times, why don’t we make a function of our own, and just call it each time whenever, we get any data through form and need to sanitize it. Let’s just create it now.
Start with ‘function’ keyword and name it as ‘sanitize’.You can name any of your own choice as this is going to be a user defined function. Within execution area of our function, we are going to call all of our three internal functions we just used. Trim, htmlspecialchars and stripslashes. Now we know that we need to supply this function a data which will be sanitized by this function, so declare an argument ‘data’, this name can be any of your own choice.
functionsanitize($data)
Now put the incoming data within first internal function trim and store it in a variable and you can keep this variable’s name same. Let’s just copy and paste it for second and copy and paste for third internal function as well. Also, as we need the data out of this function to process further let’s just return the data out of this function. So, what our function is going to do is, will take the data that is supplied to it and it will perform three internal functions on this data to sanitize it from all the unnecessary items and will return the cleaned data back to us.
functionsanitize($data){
$data = trim($data);
$data = htmlspecialchars($data);
$data = stripcslashes($data);
return $data;
}
Now instead of performing these three functions one by one we will just call our user-defined function on every input we get from our users. There we go and it will perform internal functions on this we defined in our function.
Now let’s just call sanitize for all of the user submitted form data, and let’s just store all the data one by one in variables. You can keep any name for these variables of your own choice. Username, email, password and password again and that’s it. This is enough for now.We have made our form submission and getting data process little more secure.
$name = sanitize($_POST['name']);
$username = sanitize($_POST['username']);
$email = sanitize($_POST['email']);
$password = sanitize($_POST['password']);
$password_again = sanitize($_POST['password_again']);
Display Required Field Errors& Handling Form Data: (Form filling errors)
Now we want the functionality in our form, that when a user submits the form it should display messages about any errors the user has committed with. So, for this we need to append our form a little bit and it’s really not difficult. Let’s do it.
First error that we would like togenerate if user submits the form with any of the fields left empty. As we need data of all the fields for registration process. First thing we need is an array where we can store all the errors and then we can take this array and show all the errors that might have generated. Let’s create an array ‘errors’ and it’s empty right now.
$errors = array();
Now, we need to start a conditional statement,‘if’, because we need to check whether the ‘name’ field is empty or not. It means if the form data we get has empty name field then and only then generate an error.We are going to store this error in our errors array. Also, we want the‘errors’ array as an indexed array so we leave the key area empty.
if($name == ''){
$errors[] = 'Full Name is required.';
}
This array will take care of it. Put the error as ‘Full Name is required.’ So now we have one value in our ‘errors’ array or we can say one error message has been stored.
Now move on to the next field and we need to repeat same process for this field, but as we need to generate different message for each field, we can’t make a function here. In fact we can do, but that wouldn’t be so useful as almost same amount of work we needto doin that case too. So, you should also figure out whether creating your own defined function will save your time or not. Next is username, and if this is empty we are going to storeanother error messagein our errors array. ‘User name is required’.
if($username == ''){
$errors[] = 'User Name is required';
}
We will take all of these at the bottom of our PHP code as here it will definitely throw internal error of undefined index. So, create message ‘User Name is required.’ Let’s take all of these conditional statements at the bottom of our code. Do this process for all of the required fields.
We have only one little change for the repeat password error. And that is we want this field to store error either it’s empty or it’s not equals to the password. As this field inputhas to match the password field input. So, if repeat password field is either empty or it’s not equals to password input, Repeat password is required, message will be generated.
And this is it.
if($email == ''){
$errors[] = 'Email is required';
}
if($password == ''){
$errors[] = 'Password is required.';
}
if($password_again == '' || $password != $password_again){
$errors[] = 'Repeat Password field is required and must match password.';
}
Now if any of the fieldsleft empty and form is submitted, our script will store the concerned error message in ‘errors’ array.
Now we need to display all of the errors from ‘errors’ array line by line. We will show all the messages right below the heading ‘Registration Form’ so it’s easy for the user to view them. So, let’s create this functionality now. Let’s use foreach-loop here to loop through all of the errors in‘errors’ array ‘$errors as $error’ and echo $error and append it with a br tag to show each of the errors at a separate line.
foreach($errors as $error){
echo $error, '<br>';
}
Let’s just put another small heading below here ‘All fields required’.
<h5>All Fields required.</h5>
Now it’s time to run and verify our code, so, let’s go to the browser and typein the path of our script.You can see we get some errors. First one is undefined array and second is invalid argument supplied. I hope you can understand these now. As the errors array is not available until and unless the form is submitted as we defined array within if statement, so, the first erroris justified. The second error is because the errors array is undefined until the form is submitted but we are trying to supply this undefined array to foreach-loop as an argument.
Let’s get back to the code and here we need to enclose our codein a conditional statement that if errors array is set, then and only then run this foreach-loop. Let’s take our foreach-loop in this conditional statement, and that’s it.Let’s refresh the browser and you can seeno errors at all.
if(isset($errors)){
foreach($errors as $error){
echo $error, '<br>';
}
}
Now we need to check our code further, so just type some dummy data in field‘Full Name’ and hit ‘Register’ but oh we did not change the value of action attribute of thisform. Let’s go and make it empty so it wouldn’t submit the form somewhere else as we need the data on this very page.
action=""
Now back to our browser and on correctURL and let’s run it now. Put some dummy data in only Full Name field and hit Register and there you can see lots of messages, User Name is required, Email is required, Password and Repeat password is required. So, now the user knowsall the fields he needs to fill. Now, let’s put some dummy data again and fill all the form and hit register and this time no error messages shown because of all the fields of the form were filled. One more time fill the dummy data and let’s fill password and repeat password fields differently and hit register. You can see the message Repeat Password is required, let’s change it a bit and make it more descriptive. ‘Repeat Password field is required and must match Password.’
One more thing, you might have noticed, that every time the user makes a mistake he needs to fill the form again which is really not user-friendly. With error messages user should get his form data back to the form which he entered. So, let’s write a code that will give data back to our user. Let’s create it now. It’s very simple and straight forward. In every field where we want to show the data user has already submitted, let’s put another attribute ‘value’ and here we need to start PHP. And between these tags we are going to use Ternary Operator.
Ternary Operator:
We couldn’t discuss it in our,‘if’,‘else’training session. This is just like if else, when I’ll show you how we use it, you will easily understand it. Let’s only write isset($name) this is going to check that if name is set, then put a ?(question mark) it means if the condition we just wrote, is true then return $name, and then :this workssame like else, means if the condition is false then do this and we put only ‘’ empty here. So, that’s it, just echo it out, this ternary operator will check if $name is set and if it is set, it will return $name and if variable name is not set it will return an empty string.
value="<?php echo isset($name) ? $name :''; ?>"
So, we have created the functionality to display user defined data back into the form fields. Now let’s copy this and paste in every field and change the names accordingly.
value="<?php echo isset($username) ? $username :''; ?>"
value="<?php echo isset($email) ? $email :''; ?>"
We don’t need this functionality for password and repeat password as we want our users to fill these fields again. Now check this out, refresh the browser and let’s put some dummy data again. Just keep the password and repeat password different to check the changed error message and data retrieval within the fields. And here we go the message is perfectly fine and is displayed as well as the data is served within form fields too. This is the user-friendliness we wanted. Now, just retype our password and this time match our passwords and hit register and wefind no errors here. So now we have all setup, everything is working besides just one more thingremaining. If we do not getany errors, then we would like to register this user. For this purpose let’s just create a conditional statement here. If, $errors and this is our array you remember it, just going to use a built in function count. This function counts the values in an array as we have studied it before. Let’s create the condition if the number of values in our errors array, equals to 0 (zero), means if there is no value in errors array, only then echo ‘User Registered.’ And put an else statement and here we will again store a value in errors array and that is “User cannot be registered”.
if(count($errors) == 0){
echo 'User Registered.';
} else {
$errors[] = 'User can not be registered.';
}
Now, let’s get back to the browser and put some dummy data again with different passwords and here the message again along with ‘User cannot be registered’. Now, type passwords that match and hit register and here we go ‘User Registered.’ Now our form submission is complete as we have all the necessary sanitization here, all the error messages for all the data fields and at the end we have registered our user, actually only showing the registration message right now but we will store user data in Database in an upcoming day training session. This is all for today, I hope you really enjoyed today’s training session and when you will do all such activities on your own and will see the results, it will give you a sense ofsatisfaction and confidence that you have learnt much of PHP. I’ll see you on day 8, take care.