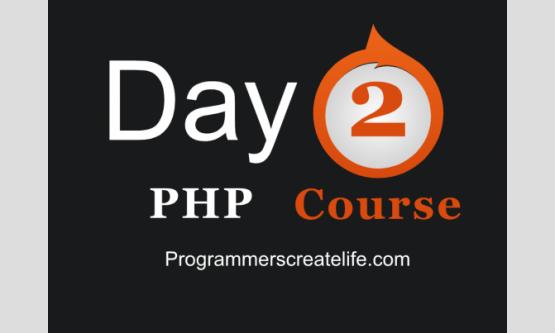
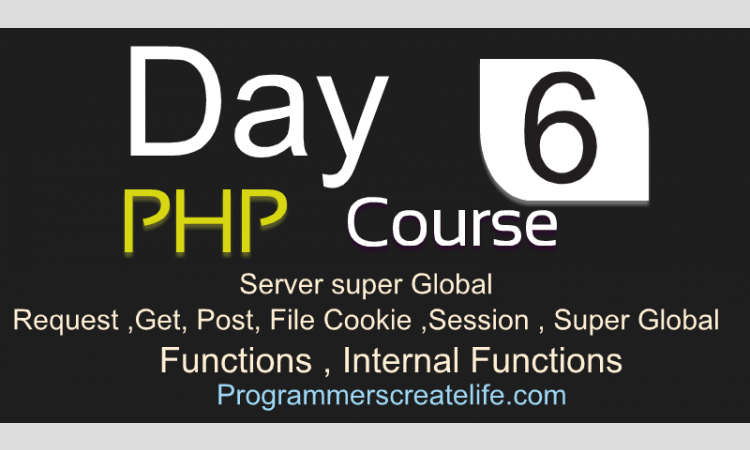
Day 6
Welcome to day6 of PHP in 15 Days – Guaranteed Training Course. Today we are going to learn
· Super-Globals
· Cookies & Sessions
· Functions
· Built In Functions
Let’s start with Super-Globals
Super-Globals:
There are many special predefined variables in PHP. These special variables are called super-globals. Specialty of these super-globals is; these are accessible at any point, within any function, regardless of their scope. You can access these variables within any function or class or anywhere. We will discuss scope of a variables later on today.
Here are the superglobals we are going to study about:
· $_SERVER
· $_REQUEST
· $_GET
· $_POST
· $_FILES
· $_COOKIE
· $_SESSION
I must add one thing more here about these special kind of variables, that these are not variables actually but arrays. Hoo? I hope you will not get confused as we have studied about arrays in detail and at this point we fully understand what these arrays are and how these work.
$_SERVER
First superglobal is $_SERVER. This superglobal is used to collect the information about http response or headers. Like you can collect the information about server name, which script is running, what is the IP of the site, IPfrom where the request or query was generated, which browser was used to access our site, what is the IP of client etc.? So, there is a lot of information you can collect using this superglobal.
I am going to show you, few of the examples like php_self, as I have already mentioned, it’s an array, so I am asking about a specific value at key php_self. This gives the information about path of the current script, which is in use. So, let’s echo it out in browser and there you can see the path information about this current script.
echo$_SERVER['PHP_SELF']; echo '<br>';
echo $_SERVER['HTTP_HOST']; echo '<br>';
echo $_SERVER['HTTP_USER_AGENT']; echo '<br>';
Let’s look at some of the others too. $_SERVER[‘http_host’] this will show you the host of the site, most probably the localhost etc.
And another one http_user_agent, this will give us the information about the browser from which our script or page was accessed. Like Mozillar, Chrome, Opera along with the nearest judged Operating system and some more information. Now, let’s view the information in our browser.
Let’s first make them line by line to make these more readable, just put br tags of html. And here we go all the information we needed. This is really not very important at this point so, just take it easy, just understand that this is an array which stores some information and you can access it anytime, anywhere you want.
$_REQUEST, $_GET, $_POST
These superglobals are used to collect form data. Whenever, we submit a form, this array is filled up and available with keys and values pairs and we can easily get the values of the form submitted. Let’s go and try to understand these.
First thing we needis to create a form which will allow us to submit some data and then we’ll write some code to grabthisdata. Let’s make our form first. For demonstration purpose I amcreating a very simple and tiny form. In the form starting tag we need to define some attributes. First attribute is method. You can choose from two types of methods i.e. Get or Post. I’lldescribe pros and cons of these in a moment. Second attribute of the form tag is action. You can keep it empty. This means, you want your form to submit data on the same page where the form is right now. Or you can just define the path of the page where you would like the data to be submitted. So, it’s up to you, you can manage it according to your own preference and requirement. For now, I want my form data on this very page. Now let’s create two fields.Username, email and we need off-coursea submit button to submit the form. We must have to declare name for each input field. These names will become the keys in our superglobal arrays and you exactly know, we grab values from an array by their keys. And that’s it, our form is ready. Let’s just put some br tags to make its display little better.
<form method="post" action="request_get_post_superglobal.php">
<br><br>
User Name: <input type="text" name="username" placeholder="User Name.."><br><br>
Email: <input type="email" name="email" placeholder="Email...."><br><br><br>
<input type="submit" value="Submit Information">
</form>
Now we are ready to submit form. Just try once again to see what we get. If we submit our form now, you can see nothing happened or changed in our browser but if you watch the address bar closely, when we clicked submit button we get some information here. And this is how the information or data passes. The names are therewhich wedefined for each name attributefor input fields but after = sign there is nothing, as we did not type anything as values. Get method will always submit form data through URL and is viewable to others. If we change the value of method attribute to post then the data submitted through this form willnot appear in URL and will not be visible to others. Let’s change methodfrom get to post and try to submit the form again.Here we go, I clicked the submit buttonbut this time no informationin the Address Bar and it seems like form was not submitted but the reality is,it’s been submitted.
Let’s change the ‘method’ back to ‘get’ and fill some dummy data in all of the fields of this form.On submitting again you can see,this time, eachvalue of the name attribute we typed in the form are again viewable, as before,along with the data we typed while filling the form is also viewable in the URL. So, this is how keys and values transmit. Also, we will use the valuesof these name attributes to grab their relevantinput.
$_REQUEST:
Now we need to grab this form data so,this is time to use our superglobal $_REQUEST array. This superglobal-array will be automatically created and available to use as soon as the form is submitted. $_REQUEST is capable to grab form data regardless of what the method has been used to submit.Keep note that $_REQUEST is a super-global array that is an array. Do you remember we created different arrays and talked about indexed and associative arrays? This $_REQUEST is actually an Associative-Array which is available on the page automatically where the form is submitted and so is super-global. The point here I want to stuff in your head is, take this array as a normal associative array. Don’t let this thing confuse you at all. So, to grab values out of this array,we will point to the keys and the keysare, we defined in the form fields. Like in the input field we defined‘username’ as name of our first form field.Let’s just put it as a key in superglobal request array here.
echo$_REQUEST['username'];
And this is it, that’s all. We have grabbed the data of username fieldof the form. Whatever the user will type we will get that. Let’s try this. Refresh the page and we get error
Undefined Index: username in C:/…………………..
Don’t worry! This is because we are echoing out a value of our superglobal request array which is not available right now.As I’ve told you before this super-global-array will be available only on the page we submit form data and only at the time when we have submitted the form. So, the time we will post or submit our form this error will disappear. We’ll learn how to fixsuch kindof errors on day7 of this training course.
Now let’s fill the form with some dummy data and try to submit it.You can see that our browser is echoing the output of the form. So, the user will submit any information through this form and we can grab it easily, using the request super-global array. Here you can see that we have the username displayed in our browser. Now,let’s do same process for the second field of the form i.e. email. And just put the same code to grab email address of the user.
echo$_REQUEST['email'];
Let’s fill some data again for name and email and just submit it. And there we go, email address. Let’s put some br tags to display the output line by line.Refresh and that’s fine.
We’ve submitted form data through get method. Now we want to use post method.There is not difference at all as far as getting the data using request super-global-array is concerned. Let’s change the value of form attribute that is method to post. Refresh the page again, errors for undefined indexes again. Don’t bother about these. Just fill out fields of the form and submit.No issues at all, we got the form data easily but only the difference you can feel that there is noany information about this data in URL as we are using post method.So, this is a secure way to submit form.
$_GET:
Now let’s try to understand get super-global, which functions same as REQUEST super-global with a little difference. Only difference is it will just grab the data if method is get. This can be useful in few of the cases to make your application little secure. This thing will enforce every input to be complaint with get-super-global. Just like $_REQUEST super-global-array, $_GET also a super-global-array i.e. an Associative-Array. So now, let’sjust change the method of our form to ‘get’ and same like before tell the keys for which the data you want to grab. Put username within square brakets and then email to get user input for both of these and just put a br tag between them to display data line by line.
echo$_GET['username'];
echo '<br>';
echo $_GET['email'];
Refresh the page and same two errors for two undefined indexes. These will disappear when we’ll submit form. Let’s fill some dummy data in both of the fields and submit. Here we go, you can see data in the browser and also, you can see it in URL too. If we change method to post, our super-global get will not be able to guess any data. It will not find any data as its just meant to grab data which is sent through get method. Let’s try to submit some data using the method post and try to grab it by get super-global. Let’s refresh the page, fill out some data here and submit, but,this time nothing has been displayed.Code is still there but nothing has been echoed. It means our code was unable tograbform data because, the data was submitted using another method which was not recognizable by $_GET.
$_POST:
Now let’s use this method for our post super-global.
echo$_POST['username'];
echo '<br>';
echo $_POST['email'];
Everything is same except the form method is changed to post, grab username and email and put a br tag between them. Now method is already post, just refresh thebrowser. Fill out some dummy data again in the form and submit. And you can see that our post super-global is grabbing the form data which is sent through post method.
Let’s play around a little bit and change method to get and try to get form data by our post super-global. But this time we already know, it will not show any data because it doesn’t recognize data sent through get method. Just refresh the page, fill some data in the form and submit. This time, you can see no data at all. Let’s change it back to post, refresh the page, fill out some data, submit and you can see the data is displayed.
You need to remember that super-global request ($_REQUEST) is used to grab form data regardless of the method used. You can use this where you want to grab each and every input.
Get super-global ($_GET) will grab only form data sent through get method and post will grab form data only sent through post method. This is all for super-global get, post and request.
$_FILES, $_COOKIE, $_SESSION (PP presentation)
The Files super-global is used to collect any file and its information that is submitted through form. We will study about this super-global array in detail on day9 of this training course.
So, let’s move on to Super-globalcookie and session.
$_COOKIE:
You might have noticed an option ‘remember me’, on most of the sites you where you login. If you check this option and login, next time you don’t have to login again.The site will let you straight into your dashboard. Sometimes you change your inbox theme to your own taste, sometimes you go to a site and it says welcome back or some greeting message specially for you, sometimes you use a site for some free stuff and then it says to register or login due to limited free attempts allowed, after some days you try to do that stuff again but still that site says you have consumed allowed free attempts. If you are a webmaster, this cookie helps you to know how many returning visitors you have on your site.These are few of many examples where cookies can be in action. Such kind of functionalities are mostly developedby cookies. Developers can also achieve these functionalities using other techniques but the easiest and simplest way is using the cookie. Cookie is a small file just like a text file. We create cookies on our own, write some information in it and set it’s time of expiry too. Afterwards, this file is sent to the client side and it remains stored in users’ browser. When user returns to our site or a page of our site, we know about this returning user by grabbing the information from that same cookie. As cookie is stored on user/client side so it’s notfoolproof way to calculate things, as users can remove these cookies at any time from history of his browser, can change the information stored etc.
Now, let’s dive into it and try to create our own cookie and store it to the client side. To create a cookie we need to write, setcookie. This is a special keyword which is used to create a cookie. After this, start parenthesis and between these parentheses we declare some parameters. First parameter is the name of this cookie.Wewill save and retrieve thiscookie from client side. Name it as you want.Let’s name it mycookie and the second parameter is the value of this cookie. This value can be anything and you will use this value for different functionalities, so, define this important factor wisely. I am just writing a string here, ‘This is value’ and third and final parameter is time of cookie life. We define it as the current time by using built in time() function. This is the current time and add seconds multiplied by minutes multiplied by hours of the day and multiplied by number of days you want to keep this cookie alive. In our case, this cookie will expire exactly after 3 days from the time it’s created.
setcookie('myCookie', 'This is the value', time()+60*60*24*3);
So, that’s it, this is how we generate a cookie and store it for some specific time on clients’ browsers. Let’s run our script now.
Go to the browser and put URL of our cookie page and enter.Our script has been run once and so, it must have created a cookie and saved it at client side. To prove that cookie has been set just go to your browser’s console and go to storage and here you can see the cookie has been stored. You can see the name myCookie and here its value we set and along with this you can see all the details of this cookie by clicking on it. Here you can see the data of this cookie. The value, creation Time, expires on and here you can see that it will expire right after 3 days. So, this cookie will live for 3 days at client side. There is other information available too like host, http only etc. So, this is howwe can generate a cookie and store it on the clients’ browser.
Now let’s grab our cookie and show its value in our browser. Let’s comment out the script which is creating cookies so that it may not overwrite the cookie we just created. To grab the cookie from client side, we use super-globalcookie array, and we put the key i.e. name of the cookie to grab a specific cookie. The name was myCookie, just write it in there and echo it out.
echo$_COOKIE['myCookie'];
So, now our browser will out put the value of ‘myCookie’ we set before ‘This is the value’ and simple is that. Let’s check it out in our browser and there we go value is shown.
So, what if we want to delete this cookie at some time. This is extremely simple and we can do it with just using setcookie built in function again and making its expiry time before the current time and keeping its value empty. So, let’s do it.
setcookie('myCookie', '', time()-100);
Let’s run our script again. And here you can seethis cookie has been deleted. If we refresh our console storage area and view it again, there is no cookie at all. So, we have deleted myCookie successfully from client side storage. Also, let’s refresh our browser page and here we are trying to echo the value of our cookie we just deleted i.e. myCookie.As we have deleted it so, here, the browser is showing error of undefined index myCookie.
So, this is howwe create cookies, store them at client side, retrieve data from these cookies anytime we want and delete them according to our own requirements. I recommend you to play around a bit with this and get used to of it and also visit php.net for more details about cookie.
$_SESSION:
Session is almost same like cookie only with few differences. Session is generated at server side and retains its state at server side.
Sessionsare mainly used to authenticate the access of users tothe private areas of our site. A very simple and general example of sessions is a login system.Login systems are fully dependent on sessions. Session is really a very secure way to check the user identity to give him different kind of permissions to let him visit secure & private areas of your site.
Insession super-global array we can generate and store as many sessions(key/value pair) as we want, and we can check their availability on all the other pages of our site according to our requirement. So, session is special super-global array which can be available at any page of our site we want.
Just keep in mind,any page where we want $_SESSION super-global array available, we need to start a session. This sounds complex but it’s really not. Let’s try this.
Don’t get overwhelmed by its name SESSION. Just believe that it’s also just an array i.e. an associative array as we discussed and proved this phenomenon for the previous super-global arrays we studied. We can store any type of data in it same like the data we store in our associative arrays.
First and the most important thing to remember and to do before working with sessions is, you must need to start the session on every page where you want to work with session super-global array. Just for an example, if you want to drive a car you must need to start it first. Same is the case with session. You need to start the session and then can get use of these sessions. How do we start a session? It’s very easy. To start a session we justissue a short and simple commandto PHP engine.Let’s write this command now;
session_start();
And that’s it. We have issued a command to PHP Engine and now we can work with sessions. Remember that this function should stay always on top. On top of everything, like headers, html head section even before defining html or any script. So, keep in mind that we must have tostart session before anything we do. Now, we are ready to work withSuper-Global Array session.
Let’s define a session now. Actually what we do is we put some data in the super-global array $_SESSION.
$_SESSION['myVar'] = 1;
myVar is key (position) and value is 1. Now let’s view this session in our browser. Just echo recently created data from our super-global array at key myVar,
echo$_SESSION['myVar'];
Let’s view it now in the browser and here we go we have 1 here. This is how we generate session variables or you may call this session only. You can store even a string as normally you store data in any associative array. Let’s change it to a string.
$_SESSION['myVar'] = 'This is session variable.';
Let’s just refresh the browser to see the change and you can see the output here, ‘This is session variable’ as we defined it.
So what we are doing here is we have an array $_SESSION and at key ‘myVar’ we have set a value ‘This is session variable.’. Again I would mention that it is as simple as we createAssociative Arrays. Just the difference is we need to start session at the top of every page like we did here by using session_start().
Now, let’s move little further. As you know $_SESSION array is a super-global, so we can access it anywhere in our whole site, on every page, in any function, class etc. So, simply if we want this array available at our cookie page, just to demonstrate you. Simply start session at the top most of the page. Let’s first comment everything here to keep things simple and clear. OK, now, just call built in function again to start the session right on top.
session_start();
Let’s come down little bit here and let’s echo session value from the session array. We just call these as sessions but you know that, this is a value of $_SESSION array. Let’s echo myVar session. Spelling and case should be correct.
echo$_SESSION['myVar'];
Let’s go to cookie_superglobal.php page and here you can see the value of session is displayed. So, this is the way we can grab all of our sessions created at any point and can easily use these for different purposes.
Now let’s create a condition on our cookie page using if. And use a built in function isset. Just to check if this session variable myVar is set or not and here if its set, just echo ‘You are allowed here’, else, means if the session variable is not set then echo ‘Not allowed here.’. This is a simple condition and let’s check it on cookie_superglobal.php page again by just refreshing it.
if(isset($_SESSION['myVar'])){
echo 'You are allowed here.';
} else {
echo 'Not allowed here.';
}
And obviously ‘You are allowed here.’ Is the message we received.The reason is we have already stored a session variable myVar, and it is set, so if condition returns true. Now, if you forget to start the session, none of the session variable will be available at that very page. Let’s comment out our call to start the session. And let’s go back to cookie page, refresh the page and you can see that ‘else’ statement executed. As because we don’t have any session variable available on this page and PHP is unable to accessthe session myVar. Although the ‘myVar’ session has been set but only available on those pages where we start the session.So, here we get the message ‘Not allowed here’.
There can be another thing, if session is correctly started but that particular session doesn’t exist or not set. For this let’s append our session file. To unset some variable, we use a built-in or internal function unset. This finds the session which we want to unset and then unsets it or you can say removes it.
unset($_SESSION['myVar']);
Just comment the script that is written to create ‘myVar’ session as this session is set now.Let’s comment this echo too. Now to unset the session ‘myVar’ first we need to run our session file as this will unset myVar. Let’s run it now. It has removed ‘myVar’ session now, it’s no longer available now.
Now to prove that our script has removed or unset the session ‘myVar’, let’s go to the cookie page. Here you can see the message ‘Not allowed here.’. Let’s go to our cookie page and try to understand what’s going on. You can see here session has been started correctly. Below here, you can see that session‘myVar’has beenverified for its existence, and because it’s been unset by running the script to unset it at session page it’s no longer available. So, the else statement executed and the message ‘Not allowed here’ displayed. Let’s try to echo our ‘myVar’ session and see what PHP engine says. This will also prove the status of our session ‘myVar’. Let’s refresh cookie page and here you can see undefined index, ‘myVar’error message. So, now it’s literally proved that ‘myVar’has been unset by our code written in session file.
Now, once again, let’s create a session‘myVar’ on session page, run our session page, here we go it’s again set and you can see its value too. Now get back to cookie page, and here the value has been displayed as well as the message, ‘You are allowed here’,because if condition returns true now.
So, this is how to start a session and get ready to work with sessions, how to create or set a session and how to grab sessions and their values at any page of our site and finally how to unset or remove a session that’s already exist.
Now, let’s take one step further and make this a little application just with few minor changes. On our cookie page if the session variable is found, show the message to the user ‘Welcome to secure area.’,and if session variable ‘myVar’ is not found then we will not allow our users to stay or even visit this page. We will redirect the users to our session page by just using a built in function.
So to do this, in the else section, except showing only the message to our users we will just call abuilt-in or an internal function and it will automatically redirect them to another page.
Call the function header, and then parenthesis and between parenthesis start quotes write LOCATION keyword and a colon and then tell the full path where you want to redirect users only if the else is executed and that’s it. That’s the way we redirect users on the occasion they are not logged in.
header('LOCATION: session_superglobal.php');
Complete Code:
if(isset($_SESSION['myVar'])){
echo 'Welcome to secure area.';
} else {
header('LOCATION: session_superglobal.php');
}
Let me elaborate everything once again, what’shappening here. First thing our script doing is, checking if something is set or not, and that something is session ‘myVar’, so, if the if condition returns true, welcome message will be displayed to our users or visitors. This means our user has permissions to visit this page because he logged in properly and assume when user logs in this session is created.Next, ifthe if condition anyway, returns false then else will be executed and here we have written the code to redirect this user to some other page and that is ‘session_superglobal.php’.
Let’s try this. This is cookie page. Just refresh and you can see welcome message as PHP finds‘myVar’ session. Let’s go to session page and you can see, myVar is available, we can see its value here. Now let’s go to session code page and comment out the session variable creation script, uncomment unset so it will unset the session variable and comment out the echo statement as well. Now let’s run session page in our browser and let it unset the session and do the necessary changes.
Now try to access cookie page type the url and when we push enter it loads the session page. Look carefully in address bar. We typed cookie page address but instead of cookie page we have been redirected to session page. This is because session variable myVar has been unset.
Now let’s change everything back to as these were before. Uncomment script that is creating the session and echo statement as well. And comment out the unset built in function so that it should not unset any session.
Now run the session page in browser again so it can create session myVar. Here you can see the value of variable. Now let’s try to access cookie page, type its path in address bar, press enter and now this time weare allowed to access it. You can see the cookie page address is there and welcome message. This is how we use sessions. We will further use this strategy when we will create login system at the end of this course.
Functions:
Functions are very interesting and useful component of any computer language. When you will learn and get used to of it you will definitely be thankful for those who developed such kind of functionality and made a coder’s life a whole lot easier.
What is a Function?
In PHPwe perform different things according to different scenarios of our project. Sometimes, we need to perform a same kind of task or a bit different task for several times at different areas of a page or on different pages of site. So, instead of copy-paste same code again and again, there is a very useful and handy thing which is called a Function.Functions not only facilitate us but also make our code look nicer, cleaner and make it more efficient. Function doesn’t only do what I told but it’s really much more than this. So, Function is defined to perform a procedure when it is called. Procedure can be a single line script only or combination of many lines of code. This code might consist of standard PHP code, functions, or anything within PHP. So, basically, function is used to perform a pre-defined procedure when we need that.
There are two types of functions.
· User Defined Functions
· Internal Functions (Built-in-Functions)
c
User Defined Functions:
At times when we are in need of some customized functionality for several times, we define a procedure of our own and keep it as a function. This type of function is calledas user-defined function. So, we have to define the whole procedure once onlyto create this function and then we keep on calling it whenever we need this. Before creating or developing a function we must have accurate procedure in mind what our function will perform. If we don’t have an idea, we cannot create one. Also, we should keep a note of thosescenarios where we would like the same function do little different action. So, we have to develop a function which basically will be a full package to deal with different scenarios and will perform the tasks accordingly.
Let’s try to makea function now.
To create a function, we need to write function keyword. This keyword tells PHP that we are going to declare a function here.Right after function keyword,we mention the name of the function. Function name can start with a small letter and an underscore which is the best practice. Each function must have a unique name. So, let’s name our first function ‘greetings’. What our function will do is, when we call it, it will output Hello World, so just echo it out in here.
functiongreetings(){
echo 'Hello World!';
}
So, this is our own user-defined-function. Let’s see it in our browser. Let’s go to our destination page, enter path in address bar, hit ‘enter’ and here you can see nothing is displayed. Nothing is here. This is because we did not call our function. Although, our function is absolutely ready but we did not call it. You can make as many functions as you want. These functions will not do anything until and unless you call them. Let’s just call it now. To call a function, we need to write its name and parenthesis and semicolon to close the line and that’s it. This is a call to ‘greetings’ function.
greetings();
Now we can view the output of our function, let’s go to the browser again, refresh and now you can see what’s expected. Our function worked and it echoed ‘Hello World!’ This is just to demonstrate you the syntax of function and how function is defined and called.
Now let’s do another thing with our function. Scroll down a bit. Now let’s create a function with the same name, we will comment out the above function, this is going to be a newer version of our function.Let’s say our function will say ‘hello James!’ when it’s called. Now, let’s first comment out our all of the above lines of code. Just keep our call to our function. You can call a function before defining it, it doesn’t matter. Only your function should not be enclosed in any conditional statement. So, we can keep this function call as the names of functionsare same.
functiongreetings(){
echo 'Hello James!';
}
Go to the browser and just refresh and here we go, ‘Hello James!’ as expected. Now, we want our function to greet some specific person to whom we want to greet when we call this function.To do this we need to pass some a value that would be the name of any person, to this function. Let’s create a variable name which will store the user defined names and we can pass it to our function. So, we need to tell our function that you will be given an argument, put $arg between parenthesis and now function can use this argument as we direct it to use.
functiongreetings($arg)
Now, we have defined that this function will get an argument then the call without this argument supplied will generate error. You can see here as well red line is indicating error.
Now let’s tell our function what to do with the argument coming to it. After echo put ‘Hello’ and concatenate it with a space as we can do easily with strings and then $arg whatever the argument will be supplied will be displayed here and then concatenate with an exclamation sign. So here we are greeting with ‘Hello’ then space and then argument which will be supplied at the time of function call.
functiongreetings($arg){
echo 'Hello'.' '. $arg.'!';
}
Now we will pass the variable ‘name’ that is storing the name of any user, to this greetings() function call here between parenthesis and it will be usedby this function. Let’s pass our variable to function, and let’s go to the browser.
greetings($name);
Here you can see an error. This is because our variable is defined after the call to the function which is absolutely wrong way to use a variable before defining it. Let’s correct it by movingthis code before the function call. Refresh the browser and now you can see the output ‘Hello James’. Now whatever the user inputs our variable will receive and that name will be greeted. Let’s change it to Mick, it will output ‘Hello Mick’ and off course if the input is a string the string will be concatenated with our Hello message. Like change it to ‘Good Morning’ and you can see in the browser ‘Hello Good Morning!’.
Sometimes it may happen that no string is available as the argument when we call our function or let’s assume our user doesn’t supply anything. In this situation only hello will be displayed. To overcome this situation as we want to greet in a good way, we can define a default value of the argument. We can assign this default value directly to our argument here in function. For this just assign $arg a value, let’s say ‘Good Morning’.
functiongreetings($arg = 'Good Morning'){
echo 'Hello'.' '. $arg.'!';
}
Now what will happen, if nothing is supplied to our function call,the function will use the default value. Let’s remove name from function call and check it out in our browser.
greetings();
Obviously the same ‘Hello Good Morning!’ message. Let’s change it to ‘Good Evening’ and just see it one more timeand once again you can see the output as expected. So now we know that default value can be given to the arguments of a function and this will be used at the time when that argument doesn’t get a value atfunction call.
Now we have default value assigned, but still we pass an argument to our function then our function will use the value supplied at the time of function call. Let’s change the value of our variable $name to Erick and obviously so, we have ‘Hello Erick’ message. Now you understand how we can supply an argument to the function and how we can set some default value for this. Now, let’s move on and do some more with functions. Comment out all of this.
Let’s create a new function ‘maths’. Now we want this function to multiply two digits and give us the result. So, let’s just echo 3 into 4 (3x4).
functionmaths(){
echo 3 * 4;
}
Very simple function. Let’s just call it and see the output.
maths();
Here we go, it’s 12.Our function is capable of multiplying two numbers and can give us the resultant. Now, let’s change our function little bit andmake this adynamic function.We want to load values dynamically through arguments. So, let’s first make variable a and give it a value 3 and another one, b and assign it value 4.
$a = 3;
$b = 4;
Now we want our function to take only those two values that we provide (arguments) instead of the values we hard quoted inside this function. So, we need to supply two arguments within parenthesis for this function. Let’s put arg1 and arg2 here. Also, instead of 3 put arg1 and instead of 4 put arg2 and this is it. We have successfully transformed our function to a dynamic function.
functionmaths($arg1, $arg2){
echo$arg1*$arg2;
}
Let’s change the values of our variable b to 5 and a to 2. Now let’s try calling our function, here we go, lots of errors. You can see ‘Missing argument 1 for maths’ , it means we did not supply argument 1 when we did call the function maths and so is the case for argument 2, undefined variables. As our function doesn’t know about these variables. Here is the missing part, let’s supply both of the arguments and put $a and $b. Now what we are doing, we are calling the function to perform the procedure we defined in it and we want to perform on the real arguments we supply it now.
maths($a, $b);
Now we have supplied both arguments and it will give us 10 as 5 into 2 results 10. Here you can see 10.
Now, we know this function call outputs some results, so we can store that result in a variable. Let’s try to store the result of the function maths in a variable C. And try to echo c, just put ‘C is: ’ and append it with our variable c. Let’s just put a line break between.
$c = maths($a, $b);
echo '<br>';
echo 'C is: ', $c;
Refresh the browser but here you can see variable c has nothing to show. It means variable c has nothing to show. It can be empty. But you can seein this code that we are assigning the result of our function to c. and echoing c right here.
$c = maths($a, $b);
echo '<br>';
echo 'C is: ', $c;
Function is working correctly as it echoes the result. We have created the variable c correctly and assigned a result of a function maths. So, what could be the problem? Something is definitely not working here. Reality is our function maths() is not capable ofgiving anything out of it. We made this function to just echo the value, so it’s echoing the result perfectly. To get a value or result out of a function we need to tell the function, please give us result out of you, so that we can use it. For this purpose we just append echo to ‘return’.
functionmaths($arg1, $arg2){
return $arg1*$arg2;
}
This return means same as it’s in English language. It will return something out of it. Now the function mathsis capable and can give us result out of it and we can use it for further processing. Now, let’s just refresh the browser and here you can see that ‘C is:’ 10. Now we can use this value as the normal variable.Let’s try to add our variable a in this value. So, a equals to 2 and c is 10 right now, the resultant should be 12.
$c = maths($a, $b);
echo '<br>';
echo 'C is: ', $c;
echo '<br>';
echo $c + $a;
On refreshing the browser, just put some br tag to show it on the next line, and here you can see 12. So, what’s happening here is that we did call our function which multiplies arg1 and arg2 and we stored the result in variable c and then we processed C just like a normal variable. This is how we can supply arguments and can process our code inside a function and can have the value out of it for further process. Now let’s comment all of this code and move ahead.
Now, let’s create our maths function again and assign somedefault values to the both arguments of this function,2 to arg1 and 4 to arg2, now let’s just create the same functionality of multiplication and return this. Now let’s just call our function without supplying anyvalues to function call and see the result in our browser, refresh, but oh we did not echo it out, so, just echo this and here the result 8 as our function used default values and performed the task.
functionmaths($arg1 = 2, $arg2 = 4){
return $arg1 * $arg2;
}
echomaths();
Now let’s just create variables a and assign value 10 and second variable b and assign value 3 and same like before supply these as values(arguments) to the function call and you can see the result 30.
echomaths($a, $b);
Now there is another thing you might sometimes in need of. We can use one value from default values and can supply one valuedynamically from any source, but it’s very important to keep in mind while doing such kind of operation, the argumentfor which you want to use the default value,must be onthe right most side.
Now let’sjust supply one value i.e. our variable b to this function which is equals to 3 and this will replace the default value for the arg1 we have supplied here. Though the second argument i.e. arg2 will use its default value.
echomaths($b);
And when we refresh the browser you can see its 12, our function multiplied variable b i.e. 3, with default value of argument 2 i.e. 4 and we got the result 12. Now, let’s change the supplied variable in our function call to variable a.
echomaths($a);
So, now we are going to multiply 10 of variable a with 4 of arg2 here and obviously the result would be 40. Here we go, refresh the browser and you can see 40 here. So, what if you want to use default value for arg1 and want to supply value for arg2, unfortunately till now this functionality is not available. As I mentioned before, you can put argument on the right most side of your arguments for which you want to use default value.
Let’s keep empty first argument and supply the value for second argument, so empty will be used for arg1 and variable a will be used for arg2,
echomaths(‘’, $a);
Let’s go to the browser and refresh and you can see that there is zero. The reason is empty was considered 0 and it’s multiplied with 10 of variable a and we got 0. Now let’s try one more time with giving null here and check it out in the browser
echomaths(null, $a);
but same result and same thing will happen if you supply 0 instead. Now let’s just change it back to variable b and refresh the browser,
echomaths($b, $a);
you can see the accurate result that is 30, a multiplied by b. So, this could have been confusing for you in future and sometimes we are desperately in need of it.For demonstration purpose at this point I think it’s enough for you. There are countless functionalitiesthat you can produce with your own functions. We will use functions in upcoming tutorials. The biggest advantage of user-defined-functions is, we can develop these to get any kind of custom functionality we need.
Built in Functions:
Think about the built in templates in your cell phone. Can you answer me what is the use of these templates?Off course these templates help you to avoid writing some frequently used texts and are ready made and ready to use. Just select one you need and just send it away. Same is the case with built in functions. These are ready made and ready to use functions which speed up your work and we don’t have to make these on our own from scratch.
As, we have already defined functions, what are these and what can these do for us. Built-in or internal function is the second type of these functions. These are used to perform only some specific pre-defined tasks and nothing more than that. So, the functions which are pre-defined, ready to use in PHP are called Built-In-Functions or internal Functions. You will be surprised to know that there are many built-in-functions you already know as we used them in this training course time to time. Now, let’s have a look atsome internal functions just to know what these do and how we can use them.
To understand these let’s create a variable ‘a’ and assign it an integer value 4. Now I want to check the type of variable a. So, we have an internal Function gettype for this purpose. Let’s use it. Gettype and put variable a in its parenthesis and let’s just echo it out so it will show us type of variable ‘a’.
echogettype($a);
That’s it. Go to the page link and hit enter and you can see the output ‘integer’ here. So, it made our work whole lot easier of getting the type of anything. We just call the function and pass it a value or variable and the function gave us the type of that variable. There is another internal function settype(). This function sets type for a variable or value or data we supply to it. Let’s use it.
settype($a, 'string');
We define two arguments here for this function call, first one is the data that is the variable ‘a’ and the second is type that we want to assign this data and that is variable ‘a’. So, you know we have assigned an integer value to variable ‘a’ so, its type is integer. But now here, with the use of this function we want to change only the type of this variable and that will be string. So, let’s inquirethe type of this variable using ‘gettype’ internal function again. Just put some br tags to make these outputs line by line.
Refresh the browser and here we go, first time its integer you can see here but after we changed its type, its ‘string’ now. We can assign any type to any data using this internal-function. Let’s make change it to float (double) data type.
settype($a, 'float');
And now it showsthe type of variable‘double’that is float, you can call it in either ways. So these are two built-in-functions to set and get types of data.
Now,let’s take a look at some of the functions which deal with strings. Strlen is an internal function to count the length of a string. This functions tells us, how many characters a string has.
echostrlen($a);
Let’s just use it. Echo it out to show what it returns and let’s just put some br tags to make these outputs line by line. So we get 1 here, it means the string we supplied consists on only 1 character and which is 4. Now make this string consisting 6 characters. And there you go it has 6 characters.
Now let’s try another internal-function substr. This function creates a sub-string from a string. Let’s create another variable ‘a’ and give it a real string, ‘This is tutorial of PHP in 15 days’. Now here in the parenthesis we need to pass few arguments, first one is the string, second one will define from where to start to creating sub-string 0 will start creating sub-string from the first letter of the string and third argument will tell at which character to stop creating the sub-string. Let’s just keep it 5 for now and echo it out and put a br tag as well.
echosubstr($a, 0, 5);
Let’s get to the browser and refresh, oh, some problem might be, let’s just make it to 10,
echosubstr($a, 0, 10);
and, oh, off course the problem is we have set its type to float.Let’s comment it out and here we go, we have created a sub string by just using an internal Function.You can change the arguments to suit your needstocreate a sub-string from a string. Let’s change it a bit and starting point is 6th Character and end point is 20the character
echosubstr($a, 6, 20);
and just check it out in the browser. There we have it.
Now I am creating an array ‘c’ and just storing some data in it.
$c = array(9, 30, 45, 23, 4);
Now let’s use print_r, another internal-function, and I guess you rememberthis very well as we have used it several times in these tutorials. So, here, just pass the array to it and it will show all the structure and values within this array. Let’s comment all the code above.
And there we go you can see in browser its showingall the values
with their detail.
print_r($c);
var_dump is another built-in-function used for almost the same purpose with little more details. Let’s try this on our array that is ‘c’.
var_dump($c);
Refresh the browser and here you can see the output.
Another built-in-function sort, already used in our previous tutorials, let’s try this on our array and then just use another internal-function var_dump to see positioning of all the values of this array. Let’s see the output and here we are, it has sorted the array easily. Let’s put br tags to make it clearer.
sort($c);
echo '<br><br><br>';
var_dump($c);
and you can see now, we have a sorted array from lowest to highest values.
Another internal function which gives us time
echotime();
Just call it as it is and no arguments required, just echo it out and we get time here. Let’s put some br tags between them. And here you can see the current time.
Another one is date and to display date we just declare format as an argument to this function. Y for year with 4 digits,m for month and d for date and you can keep any format as you like or require.
echodate("d-m-Y");
Let’s view the output in browser and there we go, let’s put some br tags again and now you can see we have displayed the date easily in our desired format. So all the internal functions are there to speed up our work and provide ready-made, ready-to-use functionality.These make a coder’s life whole lot easier. This is all internal-functions as well as for today’s training session.See you tomorrow for a new venture. Take care.
Practice files Day #6
Download